Published on Jul 26, 2023 14:51
Setting Up Your Programming Environment
Before we dive into coding with JavaScript, it's important to set up your programming environment. This will ensure that you have the necessary tools to write and test your JavaScript code effectively.
- Browser Developer Tools
Modern web browsers come equipped with built-in developer tools that are incredibly useful for writing, debugging, and testing JavaScript code. Here's how to access them on different operating systems:
- Mac: To open developer tools on Mac browsers, you can press Option + Command + I together, or you can right-click anywhere on the page and select "Inspect."
- Windows: On Windows, press Ctrl + Shift + I simultaneously, or right-click on the page and choose "Inspect."
- Linux: Similar to Windows, use the Ctrl + Shift + I shortcut, or right-click on the page and click "Inspect."
Once the developer tools are open, you'll have access to various tabs that let you inspect and modify the HTML, CSS, and JavaScript of the current web page. You can also monitor network activity and debug your JavaScript code using the console.
The image of choosing console
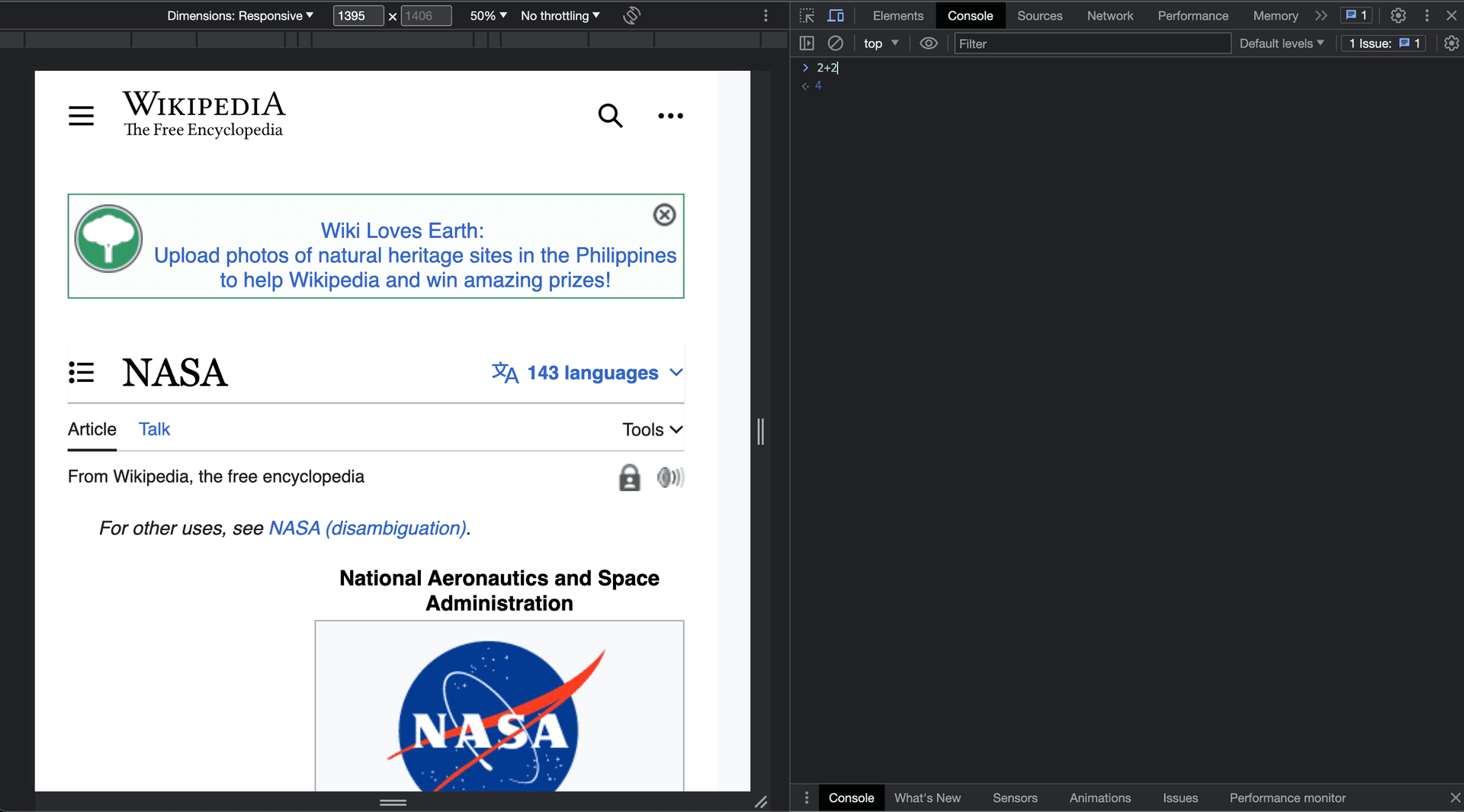
Text Editors and IDEs
For writing your JavaScript code, you'll need a text editor or an Integrated Development Environment (IDE). Here are some popular options:
- Visual Studio Code (VS Code): A widely used, free text editor. You can download it from the official website and install it according to your operating system's instructions.
- Sublime Text: Download and install Sublime Text from its official website. It's known for its simplicity and speed.
- Atom: Get Atom from the official website. It's free and highly customizable, suitable for various programming languages.
- WebStorm: For a full-featured IDE, consider WebStorm. You can download and set it up following the instructions on their official website.
Running JavaScript Code
To run JavaScript code, you can use your web browser. Here's how:
- Create an HTML file that includes your JavaScript code. You can use any text editor to do this. Try the sample below.
- Open the HTML file in your browser by double-clicking on it or using the browser's "File" menu and selecting "Open File."
- Once the page loads, right-click anywhere on the page and choose "Inspect" or "Inspect Element." This will open the developer tools.
- In the developer tools, navigate to the "Console" tab. Here, you can type or paste your JavaScript code and press Enter to run it. You'll see the output displayed right there.
Setting up your environment is the first step to start working with JavaScript. Once you're familiar with these tools, you'll be ready to write, test, and debug your JavaScript code effectively.
Try this html code below and name it index.html
<!DOCTYPE html>
<html>
<head>
<title>My JavaScript Page</title>
</head>
<body>
<script>
// Your JavaScript code here
console.log("Hello, world!");
</script>
</body>
</html>